Cooking with Flask...
Cooking up some code with Flask to demonstrate Apache load-balancing.
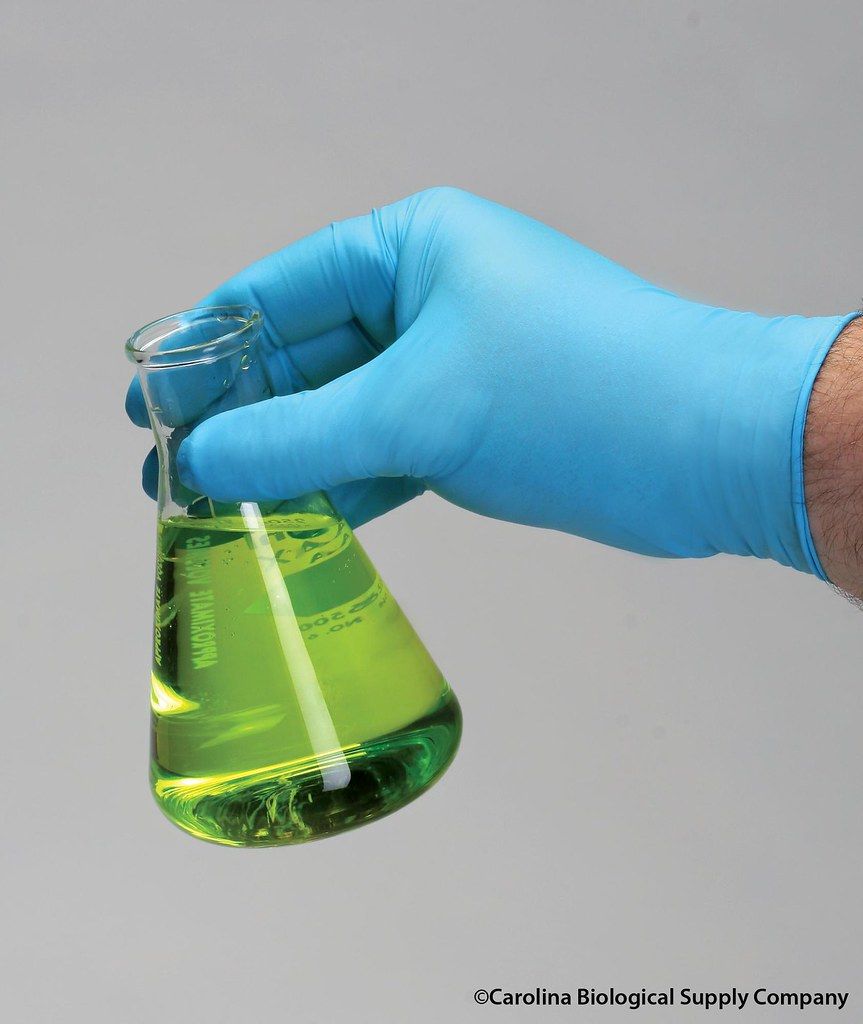
The following is a little web environment I built to test out load balancing between Flask web apps. I need to clean up the VM hosting this and didn't want to lose the activity, so here it is in a nutshell for all to enjoy and replicate as needed. I'm not going into a lot of setup details as by the time you read this (possibly even now as I write it) the precise steps would have changed. Always consult your latest documentation.
The recipe is as follows:
- Linux host (pick your Tux)
- Apache web server (v2.4)
- Python3 with Flask modules
- Balance (https://balance.inlab.net/download/)
Steps (high-level)
- Install Apache
- Install Balance module
- Configure httpd configs for Balance (see example below)
- Install Python3
- Install Flask
- Code you web apps
- Fire your web apps and see if it works!
Below are a couple of snippets from my HTTPD config file showing how the Balancer is configured and used...in this case with two nodes:
Note: I'm replacing angle brackets with regular brackets to because of how the code is rendering in my blog engine. Copy-pasters beware!
[VirtualHost *:80]
DocumentRoot /var/www/html
ServerName warybyte.com
[Proxy balancer://mycluster]
BalancerMember http://127.0.0.1:8090
BalancerMember http://127.0.0.1:8091
# Require ip 127.0.0.1
[/Proxy]
# call hello app
ProxyPass /hello http://127.0.0.1:8090/
ProxyPassReverse /hello https://127.0.0.1:8090/
# call howdy app
ProxyPass /howdy http://127.0.0.1:8091
ProxyPassReverse /howdy http://127.0.0.1:8091/
# balance main over both
ProxyPass / balancer://mycluster/
ProxyPassReverse / balancer://mycluster/
[/VirtualHost]
Below is one of the Flask apps...a very simple 'hello world' like app, nothing fancy, but lets me know what app I'm looking at when I'm testing the balancer
$ cat backend1.py
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return 'Hello world!'
$ cat backend2.py
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return 'Howdy world!'
Below is the script I wrote to fire all my Flask apps together...nifty when you have a bunch or want to start them via Cron.
$ cat fireflasks.sh
#$/bin/bash
# clean up existing apps
killall flask;
echo "Proceeding with webapp start!";
# python app1
export FLASK_APP=backend1.py;
flask run --port=8090 >/dev/null 2>&1 &
ps -elf | grep "8090";
# python app2
export FLASK_APP=backend2.py;
flask run --port=8091 >/dev/null 2>&1 &
ps -elf | grep "8091"
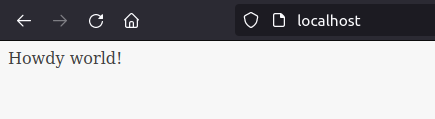
